The key moment of our rendering process is the
TGeometryArrays
class. An instance of this class
stores all the per-vertex information about the given VRML/X3D
shape. For every VRML/X3D shape, we can generate an instance of
TGeometryArrays by appropriate TArraysGenerator descendant (see
ArraysGenerator unit and ArraysGenerator function). The renderer
can use such TGeometryArrays instance to easily render the shape with
OpenGL.
TGeometryArrays stores the information about vertex positions, normal vectors, optional colors, texture coordinates (for all texture units), GLSL attributes and more. This information is split into two arrays:
one array keeps interleaved vertex positions and normals. We call it the coordinate array.
one array keeps interleaved other optional vertex data, like colors, texture coordinates, GLSL attributes etc. We call it the attribute array.
Both arrays are interleaved, allowing for fast rendering.
Separating the information into two arrays is good for dynamic shapes. When the shape coordinate changes, we have to change vertex positions and normal vectors, but the other attributes stay the same. Thanks to the fact that we have separate coordinate and attribute arrays, we can update only one of them when needed. Currently, we even have two separate VBO for coordinate and attribute arrays.
Together, the coordinate and attribute arrays describe the complete per-vertex information. TGeometryArrays.Count is the number of vertexes. TGeometryArrays.AttributeSize is the size (in bytes) of one vertex in attribute arrays, and a similar TGeometryArrays.CoordinateSize is the size of one vertex in coordinate array. Currently, coordinate arrays always stores vertex positions and normals, so CoordinateSize is actually a constant (6 * size of a single-precision float).
There is a a third, optional, array stored inside TGeometryArrays: the indexes array.
When Indexes exist, then you can render shape using glDrawElements. Each index (item on Indexes array) is an integer between 0 and TGeometryArrays.Count - 1). Indexes.Count vertexes will be drawn. A single vertex (in coordinate / attribute arrays) may be accessed many times, by using the same index many times in the Indexes array.
When Indexes do not exist, you can render using glDrawArrays. In this case, exactly TGeometryArrays.Count vertexes will be drawn.
Rendering with indexes is nice, as we conserve memory, and allow OpenGL to cache and reuse transformation and lighting calculation results for repeated indexes. Unfortunately, it's often not possible. Consider e.g. a cube with per-face normal vectors. Although you have only 8 different vertex positions, each vertex is present on 3 faces, and on each face must be rendered with different normal. This means that you have to pass to OpenGL 8 * 3 vertexes (or, equivalent, 6 * 4 = 6 faces * 4 vertexes). There's no point using indexes, and OpenGL couldn't reuse lighting calculation results anyway.
Our generator always tries to create indexes, if possible. Run
view3dscene with --debug-log, load your scene, and look for the lines
Renderer: Shape XXX is rendered with indexes:
FALSE/TRUE
in the log. This will show you how well it works
for your shapes.
For each shape that needs to be rendered, our renderer wants to generate a corresponding TGeometryArray. If an array is not created yet, a temporary generator (TArraysGenerator instance) is created, that in turn creates TGeometryArray instance corresponding to given VRML/X3D geometry.
Then the geometry array data is loaded into OpenGL vertex buffer objects. We use separate vertex buffer objects for coordinate array, attribute array and indexes array.
After loading the data to VBO (which means that the data is hopefully copied into fast GPU memory), we release the allocated memory inside TGeometryArray instance. Since that point, the data is only inside VBO, and TGeometryArray.DataFreed is true. This is a very nice memory conservation technique, the data is freed immediately after loading it to GPU. We have to keep the TGeometryArray instance (but with underlying array memory freed), as TGeometryArray knows the offsets of various attributes (colors, texture coords etc.) in the data. Effectively, TGeometryArray describes the layout of memory that is loaded into VBO.
When we detect a change to VRML/X3D model, we only regenerate and reload
to VBO needed information. For example, if you animate a shape coordinate,
we only need to reload VBO containing the coordinate array (vertex
positions and normal vectors). You can see this optimization if
you run view3dscene with --debug-log and load a model where shape coordinates
change (for example, try demo_models/x3d/worm_crawl.x3dv
).
Log lines like Renderer: Loading data to existing VBOs (1,2,3),
reloading [Coordinate]
indicate that only coordinates needed to
be reloaded.
To conserve memory usage, in case you use the same geometry many times, the process is actually a little more complicated than described in the previous section. We have a cache, that stores TGeometryArrays instance and three VBO identifiers, in a TShapeCache class. Many shapes can use the same TShapeCache instance (and thus share the same TGeometryArrays and VBO), for example when you reUSE VRML/X3D geometry, or when you have precalculated animation with the same geometry static for a number of frames. This cache allows to conserve memory and speedup rendering and loading time, in some cases making a large improvement.
If you use precalculated animation (through the
TCastlePrecalculatedAnimation
, for details see later Chapter 7, Animation) then this allows to conserve memory. The shapes that are still (or change only stuff outside of arrays/VBOs, for example only change transformation) will share the same arrays/VBO. This can be a huge memory saving, as only a single array/VBO triple may be needed for many animation frames. Very important since generating many arrays/VBOs forTCastlePrecalculatedAnimation
is generally very memory-hungry operation.For example, a robot moves by bending it's legs at the knees. But the thighs and the calves' shapes remain the same, only the transformations of the calves change.
When you have a scene that uses the same shape many times but with a different transformation. For example a forest using the same tree models scattered around. In this case all the trees can share resources, this can be a huge memory saving if we have many trees in our forest.
Figure 6.1. All the trees visible on this screenshot are actually the same tree model, only moved and rotated differently.
Note that for some features, the caching cannot
be as efficient. This includes things like Attributes.OnBeforeVertex
and the volumetric fog. In these cases, two shapes must have equal transformation
to look exactly the same. So in these cases (this is automatically
detected by the engine) we have a little less sharing,
and use more memory.
For example, look at these two trees on a scene that uses the blue volumetric fog.
Figure 6.3. The wrong rendering of the trees with volumetric fog, if we would use the same arrays/VBO (containing fog coordinate for each vertex) for both trees.
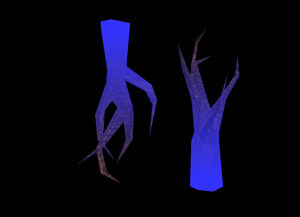